The Paradigm Shift in Service Delivery Models
Conventional service delivery frameworks are losing relevance during changing market conditions. Integrated AI represents more than just a technological elaboration; it’s a groundbreaking strategy for tackling abnormalities, overcoming scalability challenges, and fulfilling customer demand immediately.
A better visual image can be designed if we reflect on the healthcare sector, AI is optimizing workflows and enabling real-time diagnostics. Machine learning algorithms dissect patient data to predict potential health issues, permitting medical professionals to act defensively. For instance, AI models can be integrated with Electronic Health Record (EHR) systems to automatically flag malformation into the patient data, improving patient care and reducing error rates.
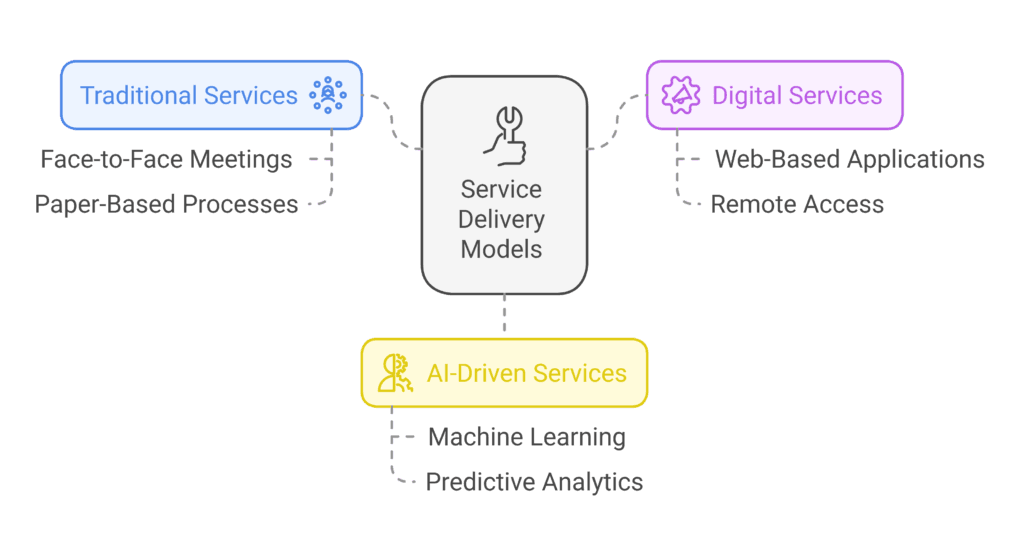
Understanding Service Delivery Models
Service delivery models are frameworks that outline how organizations provide value to their customers. These models encompass people, processes, and technology to ensure efficient service delivery.
Conventional methods often rely on manual processes and outdated technology. Today’s consumers demand speed, accuracy, and personalization — elements that traditional models struggle to deliver.
The Role of AI in Service Delivery
AI's Impact on Speed, Accuracy, and Personalization
- Speed: Automated processes significantly reduce turnaround times.
- Accuracy: AI minimizes human error by leveraging data-driven insights.
- Personalization: AI tailors services to individual customer preferences at scale.
How Integrated AI Reengineers Service Delivery Models
AI automates repetitive tasks, freeing up human resources for higher-value activities. For instance, AI-powered systems can motorize customer service through chatbots, enabling human agents to focus on more complex probes. In e-commerce, AI algorithms can operate inventory counts, process orders, and predict restocking needs, significantly reducing manual labor and human error.
We can illustrate this, In a customer service setting, AI-driven chatbots can handle common inquiries like tracking an order or processing a refund. Once a customer interacts with the bot, it autonomously processes the request and provides a response, allowing human agents to handle more intricate issues that require personal attention or decision-making.
Code Example:
import openai
import time
openai.api_key = 'your_api_key'
def automate_task(task_description):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=f"Automate the task: {task_description}",
max_tokens=100
)
return response.choices[0].text.strip()
# Example task description
task = "Process customer refund request"
# Automate the task
result = automate_task(task)
print(result)
Predictive Analytics: Anticipating Customer Needs
Code Example:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import accuracy_score
from sklearn.preprocessing import StandardScaler
# Load the dataset (replace this with your actual dataset path)
# Assuming columns: 'Age', 'BrowsingTime', 'PastPurchases', 'CustomerType', 'ProductCategory', 'LikelyToPurchase'
# You may need to load data from CSV or database
df = pd.read_csv('customer_data.csv')
# Data Preprocessing
# Convert categorical variables into dummy variables (e.g., 'CustomerType', 'ProductCategory')
df = pd.get_dummies(df, drop_first=True)
# Features (independent variables)
X = df.drop('LikelyToPurchase', axis=1) # Remove the target variable
# Target (dependent variable)
y = df['LikelyToPurchase'] # Target: Whether the customer will purchase (1) or not (0)
# Standardize the features (scaling to improve model performance)
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
# Train-test split (80% training, 20% testing)
X_train, X_test, y_train, y_test = train_test_split(X_scaled, y, test_size=0.2, random_state=42)
# Build and train the Random Forest Classifier
model = RandomForestClassifier(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
# Make predictions on the test data
y_pred = model.predict(X_test)
# Evaluate the model accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Model Accuracy: {accuracy * 100:.2f}%")
# Predicting whether a new customer will purchase based on specific input features
new_customer_data = pd.DataFrame({
'Age': [30],
'BrowsingTime': [45],
'PastPurchases': [5],
'CustomerType_Regular': [1], # Assuming the customer type is regular (1 = Regular, 0 = New)
'ProductCategory_Electronics': [1] # Assuming the product category is electronics (1 = Yes, 0 = No)
})
# Scale the new customer data using the same scaler
new_customer_scaled = scaler.transform(new_customer_data)
# Predict if the new customer will make a purchase
prediction = model.predict(new_customer_scaled)
print(f"Will the new customer purchase? {'Yes' if prediction[0] == 1 else 'No'}")
Personalization at Scale: A Tailored Experience
Code Example:
import random
# Sample customer data (this can be dynamically fetched from a database)
customer_profiles = {
'john_doe': {'preferences': ['tech', 'sports'], 'location': 'New York', 'last_login': '2024-12-15'},
'jane_smith': {'preferences': ['fashion', 'travel'], 'location': 'California', 'last_login': '2024-12-14'},
'susan_lee': {'preferences': ['cooking', 'wellness'], 'location': 'Texas', 'last_login': '2024-12-10'}
}
# Sample content based on customer interests
content_library = {
'tech': ['Latest Gadgets', 'AI Innovations', 'Tech Startups'],
'sports': ['Football Highlights', 'Basketball News', 'Olympics Updates'],
'fashion': ['Winter Fashion Trends', 'Sustainable Fashion', 'Celebrity Styles'],
'travel': ['Top Destinations for 2025', 'Budget Travel Tips', 'Luxury Resorts'],
'cooking': ['Vegan Recipes', 'Quick Dinner Ideas', 'Healthy Meal Plans'],
'wellness': ['Mindfulness Techniques', 'Home Workouts', 'Mental Health Tips']
}
# AI-powered function to personalize content
def personalize_experience(customer_id):
# Get the customer profile
profile = customer_profiles.get(customer_id)
if not profile:
return "Customer not found."
# Retrieve the customer's preferences
preferences = profile.get('preferences', [])
personalized_content = []
# Select content based on customer's preferences
for preference in preferences:
if preference in content_library:
personalized_content.append(random.choice(content_library[preference]))
# Create a personalized message
location = profile.get('location', 'Unknown')
last_login = profile.get('last_login', 'N/A')
personalized_message = f"Hello, {customer_id.capitalize()} from {location}! "
personalized_message += f"Last time you visited: {last_login}. Here are some personalized suggestions for you:\n"
personalized_message += '\n'.join(personalized_content) if personalized_content else "No content available based on your preferences."
return personalized_message
# Example usage
customer_id = 'john_doe'
print(personalize_experience(customer_id))
Enhancing Collaboration Through AI-Driven Insights
Code Example:
import random
# Sample data: Project updates from different departments
department_updates = {
'sales': {'status': 'On Track', 'progress': 85, 'issues': ['High lead churn', 'Need updated marketing material']},
'marketing': {'status': 'Delayed', 'progress': 70, 'issues': ['Resource constraint', 'Campaign approval delays']},
'product': {'status': 'On Track', 'progress': 90, 'issues': ['Bug fixes pending']},
'hr': {'status': 'On Track', 'progress': 95, 'issues': ['Onboarding new hires']},
}
# AI module to generate insights
def ai_generate_insights(updates):
insights = []
for dept, data in updates.items():
# Highlight departments with delays
if data['status'] == 'Delayed':
insights.append(f"⚠ {dept.capitalize()} is facing delays. Recommended action: Allocate more resources.")
# Highlight progress > 90%
if data['progress'] > 90:
insights.append(f" {dept.capitalize()} is nearing completion. Ensure final checks.")
# Provide recommendations for issues
for issue in data.get('issues', []):
insights.append(f" {dept.capitalize()} issue: '{issue}'. Suggested action: Review during inter-departmental meetings.")
return insights
# AI module to prioritize collaboration tasks
def ai_prioritize_tasks(updates):
task_priority = []
for dept, data in updates.items():
for issue in data.get('issues', []):
urgency = random.randint(1, 10) # Random urgency level for demonstration
task_priority.append({'department': dept, 'issue': issue, 'urgency': urgency})
# Sort tasks by urgency
return sorted(task_priority, key=lambda x: x['urgency'], reverse=True)
# Generate insights
insights = ai_generate_insights(department_updates)
print("AI-Driven Insights for Collaboration:")
print("\n".join(insights))
# Generate prioritized tasks
tasks = ai_prioritize_tasks(department_updates)
print("\nPrioritized Collaboration Tasks:")
for task in tasks:
print(f"Department: {task['department'].capitalize()}, Issue: {task['issue']}, Urgency: {task['urgency']}")
# AI-driven collaboration message
def collaboration_summary(insights):
timestamp = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
summary = f" Collaboration Summary as of {timestamp}:\n"
summary += "\n".join(insights)
return summary
# Output a summary
summary = collaboration_summary(insights)
print("\n" + summary)
Use Cases: AI-Driven Service Delivery in Action
AI upgrades scheduling and provides diagnostic support, ensuring better patient outcomes.
Success Metrics: Hospitals using AI-driven scheduling systems have observed a 25% reduction in patient wait times, At the same time symptomatic tools charged by AI have improved early detection rates of critical conditions by 35%.
Retail: Revolutionizing Customer Experience with Real-Time Suggestions
AI enhances shopping experiences through personalized product recommendations.
Success Metrics: Retailers recruiting AI-driven personalization have experienced a 30% increase in customer engagement and a 20% boost in sales.
Finance: Streamlining Loan Approvals and Fraud Detection
AI accelerates loan processing and identifies fraudulent activities with unparalleled precision.
Success Metrics: Financial institutions report a 40% depletion in loan approval time and a 50% upgrade in fraud detection authority.
Overcoming Barriers to Adoption
Clear communication and incremental performance foster smoother transitions.
Here is a record in which a global manufacturing firm faced resistance to implementing AI for predictive maintenance, they started with a pilot program in one facility. By exhibiting cost savings of 25% through reduced equipment downtime, they built trust and gained company-wide support for AI adoption.
Data Privacy and Security Concerns
Training Teams to Work with AI
Key Benefits of AI Integration in Service Delivery Models
- AI slows down operational costs by automating routine tasks and improving resource allocation.
- Personalized and optimum service delivery cultivates loyalty and repeat business.
- Organizations imposing on AI gain a strategic edge, positioning themselves as industry leaders.
Future Drifts: AI’s Growing Role in Service Delivery
- The convergence of AI with IoT and blockchain enhances data security, connectivity, and operational efficiency.
- Hyperautomation combines AI, machine learning, and robotic process automation to achieve end-to-end business transformation.
Conclusion
Brew up to upgrade your service delivery model with Integrated AI? Contact us today to start your journey toward efficiency, scalability, and unparalleled customer satisfaction!
FAQ
frequently asked & question
Integrated AI alludes to the unseamed incorporation of artificial intelligence into organizational workflows, self-regulating processes, enhancing accuracy, and enabling real-time decision-making. It reevaluates service delivery by optimizing resource allocation, streamlining operations, and providing scalable, data-driven solutions tailored to customer needs.
Orthodox models often suffer from inefficiencies, bottlenecks, and scalability limitations. AI reduces these issues by computerized decision-making, escalating communication between departments, and providing real-time intutions, deriving in faster response times, shrinked operational costs, and refined accuracy.
AI nourishes cybersecurity by recognizing anomalies, divining potential threats, and automating security patches. By amalgamting AI with existing IT infrastructures, organizations can proactively monitor systems, detect vulnerabilities, and prevent data breaches, warranting robust data protection.
AI makes even scalability by automating repetitive tasks, prophetic resource needs, and photostating dynamic workloads. Through machine learning and prophetic analytics, AI systems modifying to demand fluctuations, ensuring uninterrupted service delivery without compromising quality or performance.
Yes, AI-driven visions provide a link up view of operations across departments, bridging communication gaps and promoting data transparency. AI magnifies collaboration by automating workflows, sow the seeds of actionable insights, certifying consistent information flow, fostering aligned the inter-departmental efforts.
AI leverages data analytics to personalize customer interactions at scale. By analyzing historical data, AI anticipates customer needs, tailors recommendations, and automates service customization, thereby improving customer engagement and satisfaction.